Profile
[1]:
import svvamp
Define a random generator of profiles using the Spheroid model (which extends Impartial Culture to utilities), with 9 voters and 5 candidates:
[2]:
random_profile = svvamp.GeneratorProfileSpheroid(n_v=9, n_c=5)
random_profile
[2]:
<svvamp.preferences.generator_profile_spheroid.GeneratorProfileSpheroid at 0x227236d1310>
Use the generator to create a random profile:
[3]:
profile = random_profile()
If you wish, you can give a label to each candidate:
[4]:
profile.labels_candidates = ['Alice', 'Bob', 'Catherine', 'Dave', 'Ellen']
Basic information about the profile:
[5]:
profile.n_v
[5]:
9
[6]:
profile.n_c
[6]:
5
[7]:
profile.labels_candidates
[7]:
['Alice', 'Bob', 'Catherine', 'Dave', 'Ellen']
Voters’ rankings of preference:
[8]:
profile.preferences_rk
[8]:
array([[0, 3, 1, 2, 4],
[2, 0, 1, 3, 4],
[3, 0, 2, 1, 4],
[2, 3, 0, 4, 1],
[0, 4, 1, 2, 3],
[4, 2, 1, 3, 0],
[2, 1, 3, 0, 4],
[3, 1, 4, 2, 0],
[3, 2, 4, 0, 1]])
Voters’ utilities for the candidates:
[9]:
profile.preferences_ut
[9]:
array([[ 0.56962423, 0.23147669, -0.10420753, 0.46170276, -0.63080752],
[-0.10565873, -0.38552417, 0.22279825, -0.55394695, -0.6954934 ],
[-0.26048526, -0.43251655, -0.41065507, 0.28538753, -0.70355756],
[ 0.26890645, -0.52263056, 0.58980142, 0.55356667, 0.01564742],
[ 0.04576047, -0.46090844, -0.48370682, -0.59680883, -0.44194607],
[-0.27629285, 0.37454992, 0.44890641, 0.25299888, 0.71961741],
[-0.02726965, 0.30157615, 0.75419276, 0.13546169, -0.56670239],
[-0.13770358, 0.62540861, -0.0909895 , 0.73785947, 0.192837 ],
[-0.03753725, -0.29808471, 0.34075518, 0.88258878, 0.12107605]])
Plot the restriction of the population to 3 candidates, for example [0, 2, 3] (Alice, Catherine and Dave), in the utility space:
[10]:
profile.plot3(indexes=[0, 2, 3])
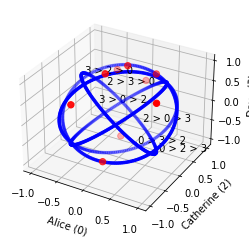
Plot the restriction of the population to 4 candidates, for example [0, 1, 2, 4] (Alice, Bob, Catherine and Ellen), in the utility space:
[11]:
profile.plot4(indexes=[0, 1, 2, 4])
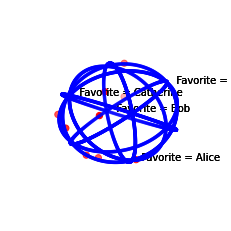
Plurality score, Borda score and total utility of each candidate:
[12]:
profile.plurality_scores_ut
[12]:
array([2, 0, 3, 3, 1])
[13]:
profile.borda_score_c_ut
[13]:
array([18., 15., 23., 22., 12.])
[14]:
profile.total_utility_c
[14]:
array([ 0.03934382, -0.56665305, 1.2668951 , 2.15881001, -1.98932905])
Matrix of duels (weighted majority graph) and matrix of victories (unweighted majority graph):
[15]:
profile.matrix_duels_ut
[15]:
array([[0, 6, 3, 3, 6],
[3, 0, 3, 4, 5],
[6, 6, 0, 5, 6],
[6, 5, 4, 0, 7],
[3, 4, 3, 2, 0]])
[16]:
profile.matrix_victories_ut_abs
[16]:
array([[0., 1., 0., 0., 1.],
[0., 0., 0., 0., 1.],
[1., 1., 0., 1., 1.],
[1., 1., 0., 0., 1.],
[0., 0., 0., 0., 0.]])
Condorcet winner:
[17]:
profile.condorcet_winner_ut_abs
[17]:
2
By convention, if there is no Condorcet winner, then SVVAMP returns nan (not a number).